Sometimes when programming you need to put a condition in to a program.
It is very similar to the IF statement in Excel.
Let us use the message box to explain the if statement
There will be two different lessons in this one as to explain the If Else statement, I need a concrete example and message boxes work well.
Sub TestConditional()
Dim answer
answer = MsgBox("Do you want to continue?", vbYesNo, "So do you?")
If answer = vbNo Then
MsgBox "You have chosen poorly"
Else
MsgBox "You have chosen wisely"
End If
End Sub
I have intentionally indented inside the IF statement to make it easier to read.
In VBA, indenting is one of the better ways to make code more legible.
The Message box has some new elements in it. I have added parameters.
vbYesNo means that the button will only have two choices, Yes or No.
The last parameter I have included is the Title in the message box, “So do you?”
When you add parameters to a message box, the result needs to be stored in a variable.
I have named my variable “answer”.
If you would like to add some kind of warning icon or ensure that the default button being highlighted is the second button, you would do this.
Sub TestConditional()
Dim answer
answer = MsgBox("Do you want to continue?", vbYesNo + vbCritical + vbDefaultButton2, "So do you?")
If answer = vbNo Then
MsgBox "You have chosen poorly"
Else
MsgBox "You have chosen wisely"
End If
End Sub
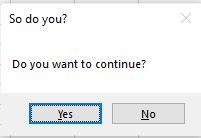
Today’s homework:
Create a sub called HomeworkConditional
Create a string variable called strAnswer input the value “Perhaps”.
Using an IF statement, check if the value in the variable strAnswer is equal to “Nope”.
If it is equal, create a message box saying “Told you so”.
If it is not equal, create a message box saying “Everybody makes a mistake”.